There can be many ways of doing it like to first create some kind of history class (just an ordinary class in dot net), expose some method of the class and then in every txtBox_Changed event or rdButton_Changed event or any other user input control event of every page, set the appropriate property on the class level domain objects based on the current value of the control being changed.
The above solution may be handy in some situation but it was not very sound enough as I was looking for a simple, generic solution that should not be heavy enough yet powerful to fit into the situation.
Background
As it can be observed from the above described problem that the solution which can play suitably in such a situation is a client side code as opposed to sever side.
So I choose to play the game in the client side with the help of java script. After all, the server side controls after render by the browser changes into the normal html controls only.
Few Key Concepts
Basically, there are a few DOM properties to be known for solving this problem and henceforth I have included this.
Property Name Description Syntax Applicable for which HTML elements
default Value The default value of a text field. The default value is the value specified in the HTML "value" attribute. When a page loads for the first time, the value of the text field is stored in this property. textField.defaultValue=somevalue Text, Text Area, Password, File, Hidden field
value The Value attribute sets the value for the input fields. The runtime change values for the input fields are stored in this attribute. inputField.value=somevalue Text, Text Area, Password, File, Hidden field,Radio Button, Checkbox, Button
defaultChecked The default value of the Check box or Radio buttons' checked attribute. The attribute proves to be true if the checkbox or radio button is checked at the time of page load i.e. default, otherwise it is false. checkboxField.defaultChecked
radiobuttonField.defaultChecked Check box, Radio button
checked The checked value of the Check box or Radio button at runtime is stored in this attribute checkboxField.checked
radiobuttonField. checked Check box, Radio button
defaultSelected The default value of the select-one or select-multiple control's. selectField.options[n]. defaultSelected Single select,
Multi select
selected The selected value of the select-one or select-multiple control's at runtime is stored in this attribute selectField.options[n].selected Single select,
Multi select
Walkthrough the code
The code is self explanatory. A small algorithm is running behind for its task
Algo Name: MonitorChangeValues
Procedure:
Step 1: Get all the elements of the form
Step 2: Loop thru the form
Step 3:
For all Text, TextArea, Password, File and Hidden fields check if the defaultValue field and the value fields are same or not. If they are unequal, this indicates that something has been changed.
For all Checkbox, RadioButton fields check if the defaultChecked field and the Checked fields are same or not. If they are unequal, this indicates that
something has been changed.
For all Select-single and Select-multiple fields check if the defaultSelected and Selected fields are same or not. If they are unequal, this indicates that something has been changed.
Step 4: If Step 3 is valid, then generate the report
The above algorithm is depicted in the following code snippet
//Function Name: CheckForModification
//Purpose: Check if anything has been changed in the form before submission
function CheckForModification(_theForm)
{
//Variables section
var changeVal="";
var _flag = 0;
//Total number of elements/controls in the form
var _totalElementsPresent = document.getElementById(_theForm).elements.length;
//Real logic
for( var i=0;i<_totalElementsPresent;i++)
{
var _formObject = document.getElementById(_theForm).elements[i];
switch(_formObject.type)
{
case 'text':
case 'textarea':
case 'password':
case 'file':
case 'hidden':
if (_formObject.value != _formObject.defaultValue)
{
if(_formObject.value != "")
{
changeVal += GenerateReport(_formObject);
_flag = 1;
}
}
break;
case 'checkbox' :
case 'radio' :
if (_formObject.checked != _formObject.defaultChecked)
{
if(_formObject.value != "")
{
changeVal += GenerateReport(_formObject);
_flag = 1;
}
}
break;
case 'select-one' :
case 'select-multiple':
for (var j = 0; j < _formObject.options.length; j++)
{
if (_formObject.options[j].selected != _formObject.options[j].defaultSelected)
{
changeVal += GenerateReport(_formObject);
_flag = 1;
}
}
break;
}
}
if(changeVal.length >0) // indicates something has been changed
document.getElementById('divDisplayStatistics').innerHTML = changeVal;
}
Real Time example
Now let's see how this can be used in the real time example. I have created a simple form with some controls.
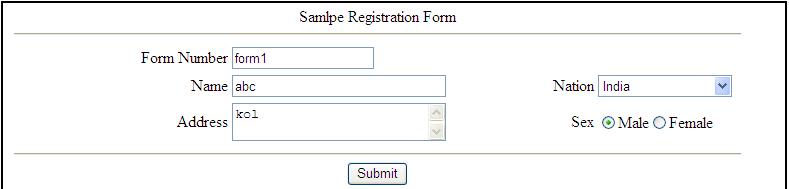
So, the "MonitorFormChangeValues.js" file is called from the Submit button client event and if any thing has been changed then it is keeping track of that by storing into the history record (a function whose signature is StoreInHistory(string strObjects)). Sometimes it is needed in projects to keep track of the changes (e.g. which control is changed,old and new values) and to store into the database.
Conclusion
It is just a way I felt easy to keep track of the changes made in the form elements at runtime.
No comments:
Post a Comment